As a tech enthusiast, serial entrepreneur, and renegade coder, I am always looking for a potential income generating project. Unfortunately, sometimes my ideas take too much time or effort to implement, so they never make it beyond inception. One of those ideas was the passive income library.
Table of Contents
The Concept
About two years ago, my girlfriend, Morgan, was student teaching. During that time, she found that one of the teachers at her school had a digital library which allowed them to manage all their own books. Of course, Morgan had taken quite an interest in it, so I figured I’d try to make one myself.
My initial idea was to create a basic desktop application which would use a barcode scanner to track books coming in and out. At the time, that seemed easy enough, so I got to work.
Prototype #1
Since we decided to make the library a Windows app, I went the Visual Studios route with my old friend C#. At first, we thought it would be cool to be able to use a barcode scanner, so I started building up that software. At least I tried. The problem was that we had purchased a keyboard wedge scanner which essentially just mimics a keyboard device. Naturally, I was a bit frustrated with the purchase, but we decided to work around it.
As far as I could tell, there was really no way to easily extract barcode data, so I designed the application around a text box. At least that way, we could still enter barcodes manually if needed.
using System; using System.Collections.Generic; using System.Text; namespace PopLibrary.Devices { /// <summary> /// Represents a barcode scanner device /// Some advice: Do not purchase this scanner unless /// you literally only want to enter barcodes into a spreadsheet /// Using this thing as been a complete and total nightmare because /// it is not HID-Scanner compliant /// The computer thinks its a keyboard and it uses pages that /// are blocked in the HIDDevice library in .NET /// As a result, you have to use RawInput to handle it which /// basically means writing threads at near C level code to /// handle the keyboard data stream and the barcode data stream /// </summary> class CortexScanner { public const UInt16 Vid = 0x05FE; public const UInt16 Pid = 0x1010; public const UInt16 UsagePage = 0x0001; public const UInt16 UsageId = 0x0006; } }
With the barcode scanner working, I was ready to develop the backend. However, we ran into another big challenge. How would the application know what book we were referencing? Clearly, we needed some way to ping a database and get the book matching the barcode. I didn’t plan to build up that resource myself. Instead, I looked to some free resources online.
I was fortunate enough to find a few resources, but they had their limitations. Most free resources had limits on the number of lookups a month, and many of them didn’t even have a complete catalog of books. At that point, I was sort of frustrated and stuck, so I stepped away from the project for a bit.
Prototype #2
Several months later, I got back to thinking about that library. That’s when the idea for the passive income library came about. I thought that maybe we could use Amazon’s database to fetch all our books. At the same time, we could be recommending books to our users. If they ever used any of our links to purchase books, we’d get a small cut. It seemed perfect, so I decided to get back to work.
Of course, the first stumbling block was navigating Amazon’s Product Advertising API. Most of their documentation was unusable, so I spent a lot of time just getting a feel for the library. At that point, I decided to start the project over using Java and JavaFX.
public void search() { // create and load default properties Properties defaultProps = new Properties(); try (FileInputStream in = new FileInputStream("src/com/cybrotronics/poplibrary/secret.key")){ defaultProps.load(in); } catch (IOException e) { e.printStackTrace(); } String awsAccessKey = defaultProps.getProperty("awsAccessKey"); String awsSecretAccessKey = defaultProps.getProperty("awsSecretAccessKey"); String awsAssociateTag = defaultProps.getProperty("awsAssociateTag"); // Set the service: AWSECommerceService service = new AWSECommerceService(); service.setHandlerResolver(new AwsHandlerResolver(awsSecretAccessKey)); // Set the service port: com.ECS.client.jax.AWSECommerceServicePortType port = service.getAWSECommerceServicePort(); // Get the operation object: ItemSearchRequest itemRequest = new ItemSearchRequest(); // Fill in the request object: itemRequest.setSearchIndex("Books"); itemRequest.setKeywords(searchBar.getText()); itemRequest.getResponseGroup().add("Large"); ItemSearch ItemElement= new ItemSearch(); ItemElement.setAWSAccessKeyId(awsAccessKey); ItemElement.setAssociateTag(awsAssociateTag); ItemElement.getRequest().add(itemRequest); // Call the Web service operation and store the response in the response object: bookList.getItems().clear(); ItemSearchResponse response = port.itemSearch(ItemElement); response.getItems() .forEach(itemList -> itemList.getItem() .forEach(item -> bookList.getItems().add(item))); }
Everything was going great until I accidentally committed my Amazon keys to GitHub. Almost immediately I received an email warning me of my mistake. That’s when I realized that these keys were going to be an issue.
I searched all over the internet for some way to hide these keys on the client. Most people just said not to do it as it would be nearly impossible to hide them, so I ended up at a standstill again. I just had no idea how I was going to make all this work, so I stepped away from the project again.
Prototype #3
The third and final prototype came along a couple months later when I was dabbling with WordPress. I thought maybe it would be possible to use WordPress to build up a safe web-based passive income library. After some research, it seemed a bit too difficult, so I started from scratch again.
This time I picked up my first web framework: Laravel. At the time, I knew effectively nothing about web programming, and I can’t say I understand much more now. Regardless, I decided to follow the Laracasts closely while adding in my own little features.
<?php /* |-------------------------------------------------------------------------- | Web Routes |-------------------------------------------------------------------------- | | Here is where you can register web routes for your application. These | routes are loaded by the RouteServiceProvider within a group which | contains the "web" middleware group. Now create something great! | */ Route::get('/books', 'BooksController@index'); Route::get('/books/{book}', 'BooksController@show')->where('book', '[0-9]+'); Route::get('/books/create', 'BooksController@create'); Route::post('/books', 'BooksController@store'); Route::get('/', 'HomeController@index'); Auth::routes(); Route::get('/home', 'HomeController@index');
At this point, I was dreaming of way more features than before. I wanted the website to almost be a social network of book sharing, so I used Laravel to build up the infrastructure on the backend. Users could have their own books, but they could also lend their books and borrow books from others. It was going to be a huge network of book sharing that would essentially drive sales to Amazon. From there, I would make a commission.
I remember setting up a lot of the infrastructure, but I got frustrated again with the new scope and my inability to understand web programming. There was just too much of a skill gap for me to do this project on my own, so I eventually threw in the towel.
Going Forward
I had no intentions of giving up on this project, but its the type of thing that I would probably need to be really passionate about to get done. As much as I love the idea of passive income, I could never get passed all the challenges during implementation.
That’s why I decided to share my idea with the world. Maybe a project like this already exists, and someone is making money off of it. That would be a huge relief, and I could put the project behind me.
However, if this project doesn’t exist, maybe one of my readers can help me turn it into a reality. In fact, I already have a few domains purchased to host the tool if someone just wants to help me get the library off the ground. The source code isn’t hidden at all. You can find all my efforts in the PopLibrary repo on my GitHub.
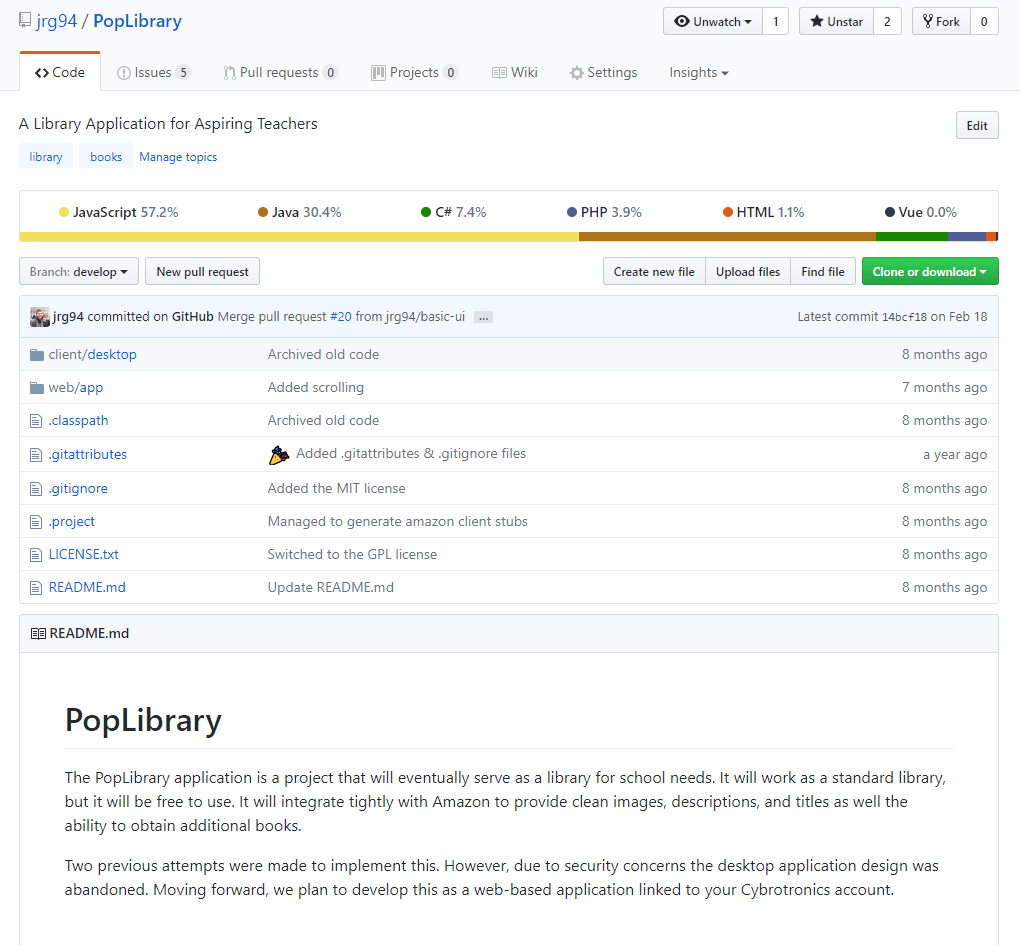
Go ahead! Fork the repo. I’d like to see if anyone can get this working.
Recent Posts
The infamous "name a woman" challenge has expanded into a speed run where you name 100 women. I took the challenge and failed miserably (hence why I'm hiding it behind the paywall).
It's July 2024, and I have three chapters of my dissertation drafted! Two more and we'll be ready to defend.