Today, we’ll take a look at a few different ways to convert an integer to a string in Python. As it turns out, each solution takes just a couple characters.
In general, if you’re looking to convert an integer to a string, you’ll want to use the string constructor as follows: str(5)
. Alternatively, you can take advantage of some of the string formatting tricks like f-strings, f"{5}"
, or string interpolation, "%s" % 5
.
If you’re looking for a few more details, keep reading!
Table of Contents
Problem Description
As you may already know, Python has a strong typing system. Typically, this term isn’t well defined, so I’ll argue that the strength of a typing system depends on how easy it is to coerce a value between types. For example, in Python, it’s not possible to treat an integer like a string without generating a separate object in the process:
"It's " + 32 + " degrees! That's cold." # ERROR!
Naturally, something like this fails because concatenation between strings expects strings. If one of the values is not a string, the concatenation fails and returns an error.
Of course, this issue comes up in many different contexts. For example, we might be trying to create a series of numbered file paths. If we do this using a loop and a counter, we’ll get an error. After all, the path constructor expects strings:
from pathlib import Path for i in range(5): p = Path("path", "to", "folder", i) # ERROR!
To solve both of these problems, we would have to convert our integer into a string first. So, how would we go about it? That’s the topic of this article!
Solutions
Given this problem, the solutions happen to be pretty straightforward. As a result, I’ll spend a little more time providing some context for each solution. As always, here’s a quick overview of what you’re getting yourself into:
- Type Casting:
str(5)
- f-Strings:
f"{5}"
- String Interpolation:
"%s" % 5
With that out of the way, let’s get started.
Convert an Integer to a String Using Type Casting
Typically, whenever we want to convert a data type from one form to another, we use a type cast. In Python, type casting is done through constructors of which there are at least three: int()
, float()
, and str()
.
Naturally, each of these functions serves to generate an object of a given type based on some input. For example, we can pass a float to int()
to get the truncated version of that value. Similarly, we can pass an integer to str()
to get the string version of that value:
str(5) # returns "5"
Depending on the context, this is probably the most common way to generate a string from an integer. For example, we might have a function which expects an integer in string format. Naturally, we would need to convert it to a string first:
some_function(str(5))
That said, often the only reason we need to convert an integer to a string is to be able to concatenate it to a string. For example, we might want to insert a number in the middle of a sentence:
"I am " + 26 + " years old" # ERROR!
Unfortunately, this causes an error because we have to cast the value first:
"I am " + str(26) + " years old" # returns "I am 26 years old"
To make matter worse, concatenation is generally frowned upon. Fortunately, there are a few other solutions to help us out!
Convert an Integer to a String Using f-Strings
In Python 3.6, the dev team introduced f-strings which are basically fancy strings that let you embed expressions in them. When the string is evaluated, the expressions are computed and converted to strings. For example, we might embed an integer in one to see what happens:
f"{5}" # returns "5"
Of course, f-strings have a lot more power than being able to convert integers to strings. We can also generate the same string above in a much cleaner way:
f"I am {26} years old" # returns "I am 26 years old"
If you’re interested in other ways f-strings can be used, check out my article on how to format strings in Python. Otherwise, let’s keep moving!
Convert an Integer to a String Using String Interpolation
If for some reason you’re a bit behind the times, no worries! Python has a ton of different ways to format strings and ultimately convert integers to strings in the process. For example, Python includes the string interpolation operator, “%”, which allows us to embed values in a string much like f-strings. In this case, we can convert an integer to a string as follows:
"%s" % 5 # returns "5"
Likewise, we can replicate the f-string code above as follows:
"I am %s years old" % 26 # returns "I am 26 years old"
Naturally, this is slightly less readable than an f-string, but it gets the job done. And, this surely beats concatenation.
That said, we’ve started to stray a bit from the initial problem and instead moved into string formatting. If you’re interested in learning more about the different ways in which we can format strings, I have another article on that. Otherwise, let’s discuss performance.
Performance
If it’s your first time checking out one of the articles in this series, then you’ll be happy to know that I always like to take a moment to compare the performance of each solution. Certainly, I don’t claim to be an expert on performance testing, but I do try to give each solution a fair assessment. If you’re interested in learning about my process, check out this article. Otherwise, let’s get started.
First, I like to store all of our solutions in strings. Given that we’re working with nested strings, we have to be careful. That said, this is what I came up with:
type_casting = """ str(5) """ f_string = """ f'{5}' """ interpolation = """ '%s' % 5 """
With our strings ready, it’s just a matter of importing the timeit
library and kicking things off:
>>> import timeit >>> min(timeit.repeat(stmt=type_casting)) 0.13226859999997487 >>> min(timeit.repeat(stmt=f_string)) 0.07017450000000736 >>> min(timeit.repeat(stmt=interpolation)) 0.11642610000001241
One of the things that always strikes me when I perform this kind of testing is how performant newer features are. As it turns out, f-strings are really, really fast. In this case, it appears they’re nearly twice as fast as the typical solution to this kind of problem: type casting.
For fun, I reran this test using a much larger integer:
>>> setup = """ number = 1234567890 """ >>> type_casting = """ str(number) """ >>> f_string = """ f'{number}' """ >>> interpolation = """ '%s' % number """ >>> min(timeit.repeat(stmt=type_casting, setup=setup)) 0.1557746999999381 >>> min(timeit.repeat(stmt=f_string, setup=setup)) 0.08932579999998325 >>> min(timeit.repeat(stmt=interpolation, setup=setup)) 0.12929110000004584
And again, with an even larger integer:
>>> setup = """ number = 12345678901234567890 """ >>> min(timeit.repeat(stmt=type_casting, setup=setup)) 0.17987480000010692 >>> min(timeit.repeat(stmt=f_string, setup=setup)) 0.11137680000001637 >>> min(timeit.repeat(stmt=interpolation, setup=setup)) 0.15611420000004728
In general, it seems the distance between each solution stays the same which suggests that f-strings lack some type of overhead. I’d be interested in digging in to see exactly what’s causing the difference, but this is where we’ll stop for today.
If you’re interested, I’ve more recently started automating all of this testing in the How to Python repo. As a result, you can find a quick overview of how these solutions compare in the following data visualization:
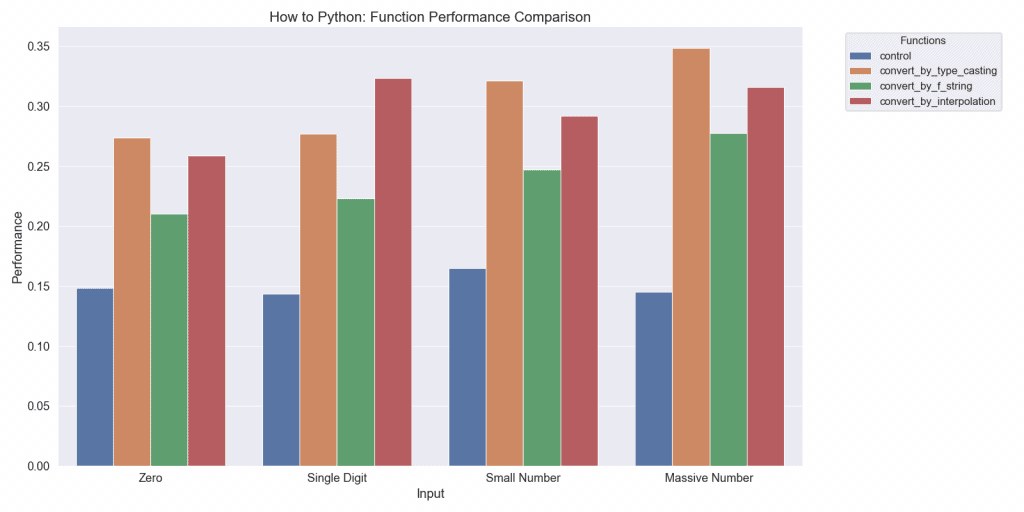
For reference, I’m running the latest version of Python at the time of writing (Python 3.9.x) on a Windows 10 machine. Your mileage may vary.
Challenge
As with any article in this series, I wouldn’t let you leave without taking your new knowledge for a spin with a challenge. Now that you know how to convert an integer to a string, let’s see if you can take it one step further.
Write a code snippet that can generate the reverse of an integer as a string. For example, give a number like 5372, I would expect the program to produce the following string “2735”.
When you think you have a solution, head on over to Twitter and dump your solution under #RenegadePython. Alternatively, you can share your solution with us on GitHub
. As always, here’s mine!
With that out of the way, let’s move on to the final recap.
A Little Recap
As always, let’s take a moment to recap the solutions in this article:
# Type cast str(5) # f-string f"{5}" # String interpolation "%s" % 5
With that said, that’s all I have for you today. If you have a moment, I’d appreciate it if you checked out my list of ways to grow the site. Over there, you’ll find links to my YouTube channel, community Discord, and newsletter.
Alternatively, you can keep reading about Python here:
- Python 3.9 Features That Will Make Your Life Easier
- How to Swap Variables in Python: Temporary Variables and Iterable Unpacking
And, you can even check out some of these references from the folks at Amazon (ad):
- Effective Python: 90 Specific Ways to Write Better Python
- Python Tricks: A Buffet of Awesome Python Features
- Python Programming: An Introduction to Computer Science
Otherwise, I appreciate you taking the time to check out the site. Come back soon!
Recent Code Posts
In growing the Python concept map, I thought I'd take today to cover the concept of special methods as their called in the documentation. However, you may have heard them called magic methods or even...
Today, we're expanding our new concept series by building on the concept of an iterable. Specifically, we'll be looking at a feature of iterables called iterable unpacking. Let's get into it!